Simple Animation Controller with Timeline
Simple lightweight library for high performance animation in a web page, makes preparations for each frame and calls the frames with optimized timeline for animation.
For example, all positions of the red arrow below in each frame are calculated beforehand, and it is moved to those positions in each frame.
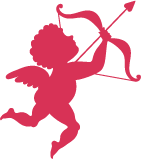

Also, AnimSequence allows the animation to synchronize with mouse-dragging. These animations are controlled by mouse-dragging event of PlainDraggable.
Note that the speed of the red arrow above and the motorcycle below changes even if the each blue pointer is moved at a constant speed.
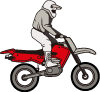
Methods
AnimSequence.add
animId = AnimSequence.add(valueCallback, frameCallback, duration, count, timing, reverse, timeRatio)
Add new animation, and return animId
that is used to start and remove the animation.
For example:
animId = AnimSequence.add(
function(outputRatio) {
return 80 + (680 - 80) * outputRatio + 'px';
},
function(value) {
element.style.left = value;
},
3000, 0, 'ease-in-out');
Arguments:
valueCallback
Type: function or undefined
Default: undefined
value = valueCallback(outputRatio)
A function that returns a value that is used to draw each frame.
For high performance animation, the function is called for all frames before the animation starts. That is, it makes preparations for each value that is used to draw the frame.
outputRatio
is a number ranging from 0
to 1
to calculate a value.
The function can return something that is used to draw an frame. The value can be an Object or Array that contains multiple values.
For example, move an element rightward, it changes a left
CSS property, from 80px
to 680px
.
// Return `80px` when `outputRatio` is `0%` (0), `680px` when `outputRatio` is `100%` (1).
function(outputRatio) {
// This value is used by `frameCallback` to draw a frame.
return 80 + (680 - 80) * outputRatio + 'px';
}
frameCallback
Type: function
toNext = frameCallback(value, finish, timeRatio, outputRatio)
A function that draws a frame.
The function is called when it should draw a frame, it draws a frame with passed arguments.
value
is something that was returned byvalueCallback
.finish
istrue
if the function should draw a last frame.timeRatio
is a number ranging from0
to1
to indicate the progress time of the animation played. It means also the ratio of the progress time andduration
.outputRatio
is the same as an argument ofvalueCallback
.
If the function returns false
, stop the animation forcedly.
For example, move an element rightward, it changes a left
CSS property.
function(value, finish, timeRatio, outputRatio) {
element.style.left = value; // It was already calculated by `valueCallback`.
if (finish) {
element.style.backgroundColor = 'red'; // Change the color to red at the right.
}
}
duration
Type: number
A number determining how long (milliseconds) the animation will run.
count
Type: number
Default: 0
A number of times the animation should repeat. The animation will repeat forever if 0
is specified.
timing
Type: Array or string
An Array that is [x1, y1, x2, y2]
as a "timing function" that indicates how to change the speed. It works same as that of CSS animation.
You can specify one of the following keywords also. These values mean keywords for common timing functions.
ease
linear
ease-in
ease-out
ease-in-out
reverse
Type: boolean
Default: false
The animation plays backward if true
is specified.
It can be specified by AnimSequence.start
also.
timeRatio
Type: number or boolean
Default: 0
A number ranging from 0
to 1
to play from the midst or false
that prevents it starting.
It can be specified by AnimSequence.start
also.
AnimSequence.remove
AnimSequence.remove(animId)
Remove an animation.
Arguments:
animId
Type: number
The animId
that was returned by AnimSequence.add
.
AnimSequence.start
AnimSequence.start(animId, reverse, timeRatio)
Start an animation.
Arguments:
animId
Type: number
The animId
that was returned by AnimSequence.add
.
reverse
Type: boolean
Default: false
The animation plays backward if true
is specified.
timeRatio
Type: number
Default: 0
A number ranging from 0
to 1
to play from the midst.
AnimSequence.stop
timeRatio = AnimSequence.stop(animId, getTimeRatioByFrame)
Stop an animation.timeRatio
is a number ranging from 0
to 1
for restarting the animation from the frame that was stopped.
Arguments:
animId
Type: number
The animId
that was returned by AnimSequence.add
.
getTimeRatioByFrame
Type: boolean
Default: false
If true
is specified, return timeRatio
of the last played frame.
AnimSequence.validTiming
isValid = AnimSequence.validTiming(timing)
Check whether an Array or string as timing
is valid.